MongoDB Cheat Sheet
Working with a MongoDB NoSQL database? This guide shows the most common functions: queries, operators, and commands.
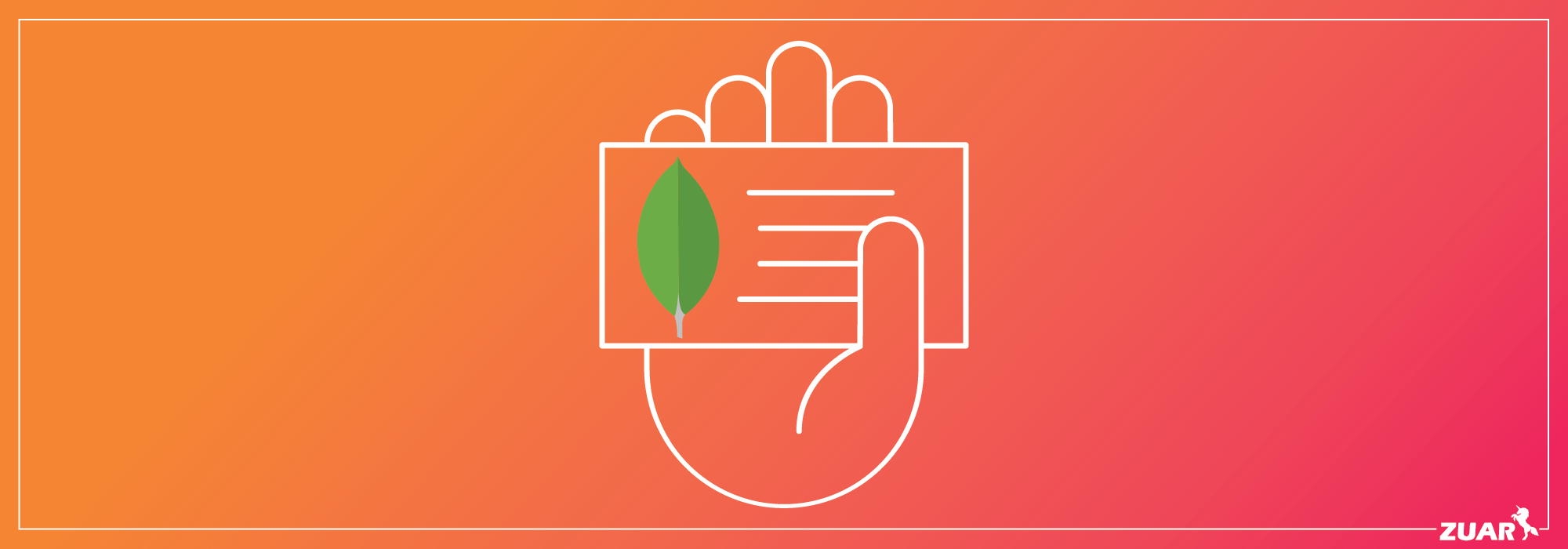
MongoDB is a flexible, document-orientated, NoSQL database program that can scale to any enterprise volume without compromising search performance. MongoDB also integrates easily with ETL/data staging platforms like Zuar Runner.
Need a quick reference cheat sheet for common MongoDB commands? Whether you’re just learning or need a refresher, we’ve got you covered.
Related Article:
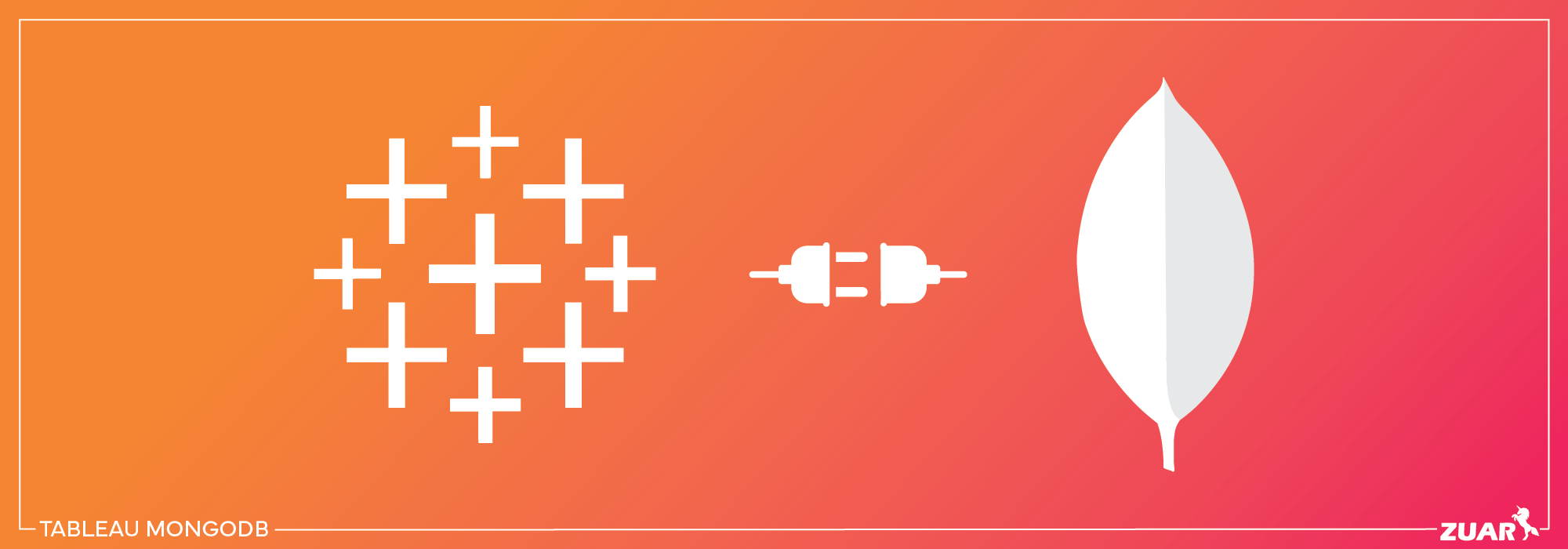
Initiation & Creation Commands
Below are commands that relate to initiating and creating a database. These are commands that are essential when you’re beginning.
Starting and Stopping the MongoDB Database:
sudo service mongod start
sudo service mongod stop
Accessing the MongoDB With Shell:
mongo --host localhost:27017
Showing All Databases:
Show dbs
Showing the Current Database:
db
Switching to a Different Database or Creating a Database:
use nameofdb
Need to improve your data strategy? Learn how Zuar can customize data strategy and solutions to meet your organization’s growing data needs.
Collection & Record Commands
These are the basic commands for collections and records in a MongoDB. Whether you’re new to MongoDB or it’s been awhile, these are your bread and butter commands.
Creating a Collection:
db.createCollection("collectionname")
Dropping a Collection:
db.collectionname.drop()
Showing All Collections in a Database:
NOTE: You’ll need to execute the “use dbname” command before you can run this command.
show collections
show tables
Or you can use this code:
db.getCollectionNames()
Inserting a Record into a Collection:
db.collectionname.insert({"field1: "content", "field2": "morecontent", "field3": "moredata"})
Updating a Record in a Collection:
NOTE: This will only update the first match, and not for duplicates. Anything not specified will be deleted from the record.
db.collectionname.update({"field1": "content"}, {field1: "updateddata”})
Deleting a Record:
db.collectionname.remove({"field1": "content”})
Deleting ALL the Records in a Collection:
db.collectionname.remove({ })
Counting the Records in a Collection:
db.collectionname.find().count()
Counting the Records by a Specific Field Value
db.collectionname.find({ field1: 'content' }).count()
Listing a Collection’s Records:
db.collectionname.find()
db.collectionname.find().pretty()
Listing Records with Matching Values of Specific Fields:
db. collectionname.find({"field1": "matchers"})
Multiple Matching Values:
db. collectionname.find({"field1": "matchers", "field2": "secondmatchingvalue"})
Finding a Single Record:
db. collectionname.findOne({"field1": "content"})
Does your organization need data staging expertise? Zuar's technical consultants can make your life easier.
Aggregation Operations for MongoDB
Common operations you’ll need for filtering and aggregating records within a collection.
Aggregating an Expression:
db.collectionname.aggregate([{$group : {_id : "$operator", num_of_records : {$sum : 1}}}])
Key Aggregation Filtering Operations:
- $project: Changes keys and values of a set of records.
- $match: Reduces the number of records for the next stage.
- $group: Groups records by one or two keys.
- $sort: Sorts the records for the next stage.
- $skip: Skips forward a specified number of records.
- $limit: Limits the number of records to search through.
- $unwind: Unwinds records that are using arrays.
- $all: finds all records that belong to the array.
Using Greater Than or Less Than:
- $gt: Greater Than
- $gte: Greater Than or Equal
- $lt: Lesser Than
- $lte: Lesser Than or Equal
- $eq: Equal
Examples:
db.collectionname.find({ views: { $gt: 2 } })
db.collectionname.find({ views: { $gte: 2 } })
db.collectionname.find({ views: { $lt: 2 } })
db.collectionname.find({ views: { $lte: 2 } })
Want to simplify and automate your data management? Contact Zuar to learn how they can help you refine your data strategy.
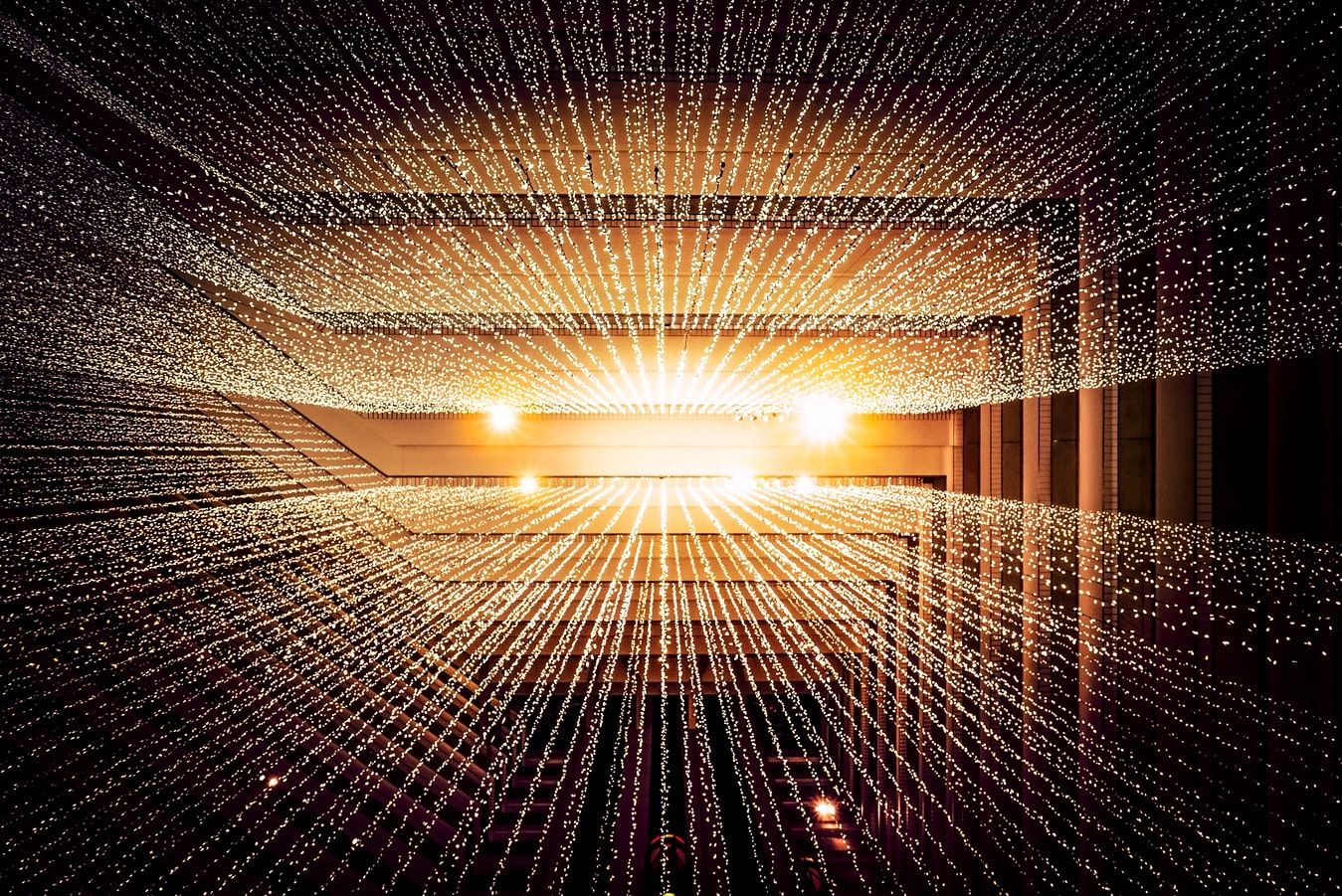
Miscellaneous Commands
Here are a few helpful miscellaneous commands that you’ll need to define your MongoDB.
Creating an Index:
db.collectionname.createIndex({ title: 'text' })
Listing All Indexes:
db.collectionname.getIndexes()
db.collectionname.getIndexKeys()
Hiding and Unhiding Indexes:
db.collectionname.hideIndex(“indexname”)
db.collectionname.unhideIndex(“indexname”)
Creating a User:
Providing the user a username, password, and database rights.
db.createUser({"user": "username", "pwd": "password", "roles": ["readWrite", "dbAdmin"]})
Showing Users for a Database:
show users
Searching Text Within a Collection:
db.collectionname.find({ $text: { $search: "\"Post O\"" } })
Finding by an Element in an Array:
db.collectionname.find({ comments: { $elemMatch: {field: 'data'}}})
Running a JavaScript File:
load(“myScript.js”)
Need to define a more efficient data strategy? Learn how Zuar’s Runner data staging platform can integrate data from multiple sources into your MongoDB.
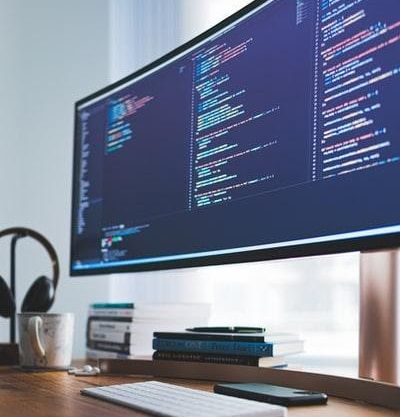
When to Use MongoDB
Backing things up a bit, let’s take a quick look at why and when you’ll want to utilize MongoDB.
MongoDB is an excellent database when you are building scalable apps that utilize Agile methodologies. MongoDB allows the developer to:
- Store and retrieve documents faster than SQL and MySQL.
- Support massive volumes of data and traffic.
- Install and code the open-source database immediately.
- Leverage the MongoDB ecosystem and community for support and troubleshooting.
- Integrate with most computing platforms regardless of whether they are in the cloud or on-premise.
- Support all major languages.
- Access the database from all major ETL and data management systems.
- Support large enterprise volume and traffic.
Some practical uses for MongoDB include:
- Supporting rapid iterative development.
- Collaborating with a large number of teams.
- Scaling to meet high levels of read-and-write traffic.
- Enable your data repository to grow to a massive size.
More on why to use MongoDB and when to use it HERE.
Check out some of our other database cheat sheets:
Taking the Next Step
If you are in the process of integrating a MongoDB into your data strategy, Zuar can assess your current system for ways to improve efficiency and automate data processes.
Zuar specializes in data management, and offers efficient products and services that automate and transform your data performance and strategy. Learn how Zuar can automate your ETL processes, enabling clean data to flow into a single destination for analytics.
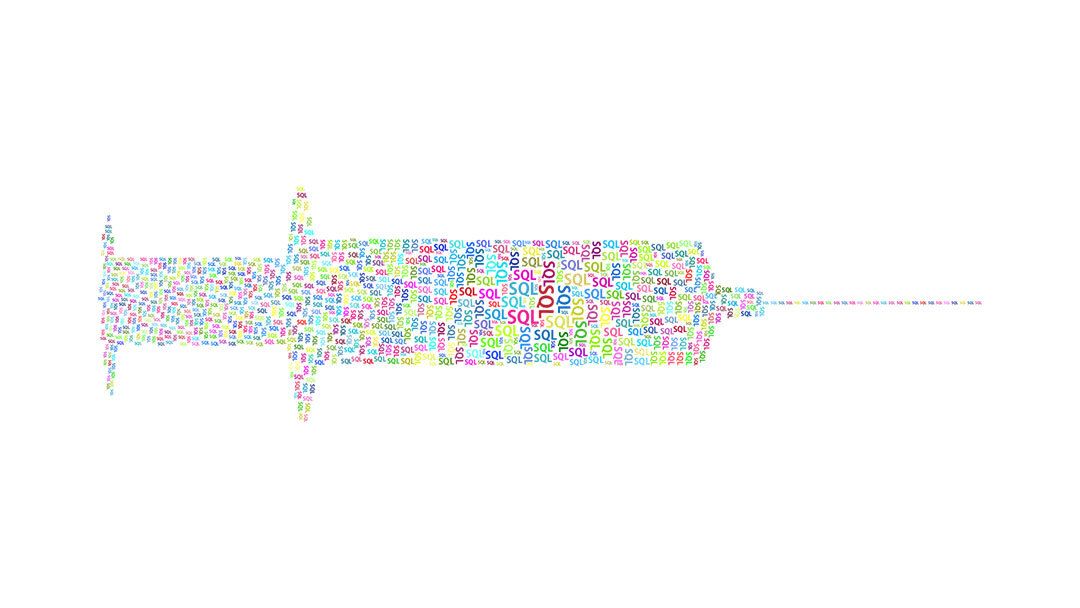
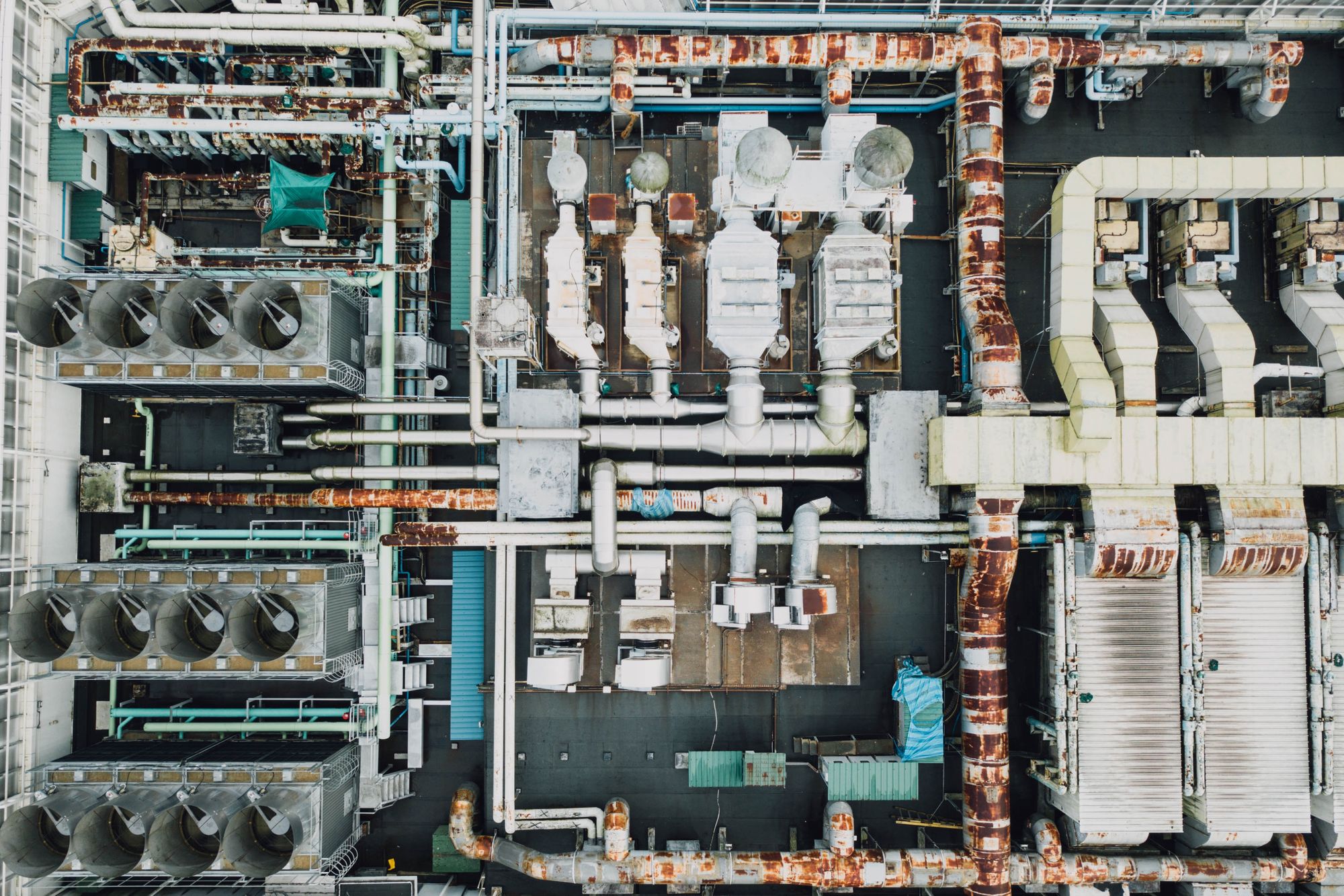