Automate the Execution of Python Scripts With Cron
Learn how to automate Python scripts via cron jobs.
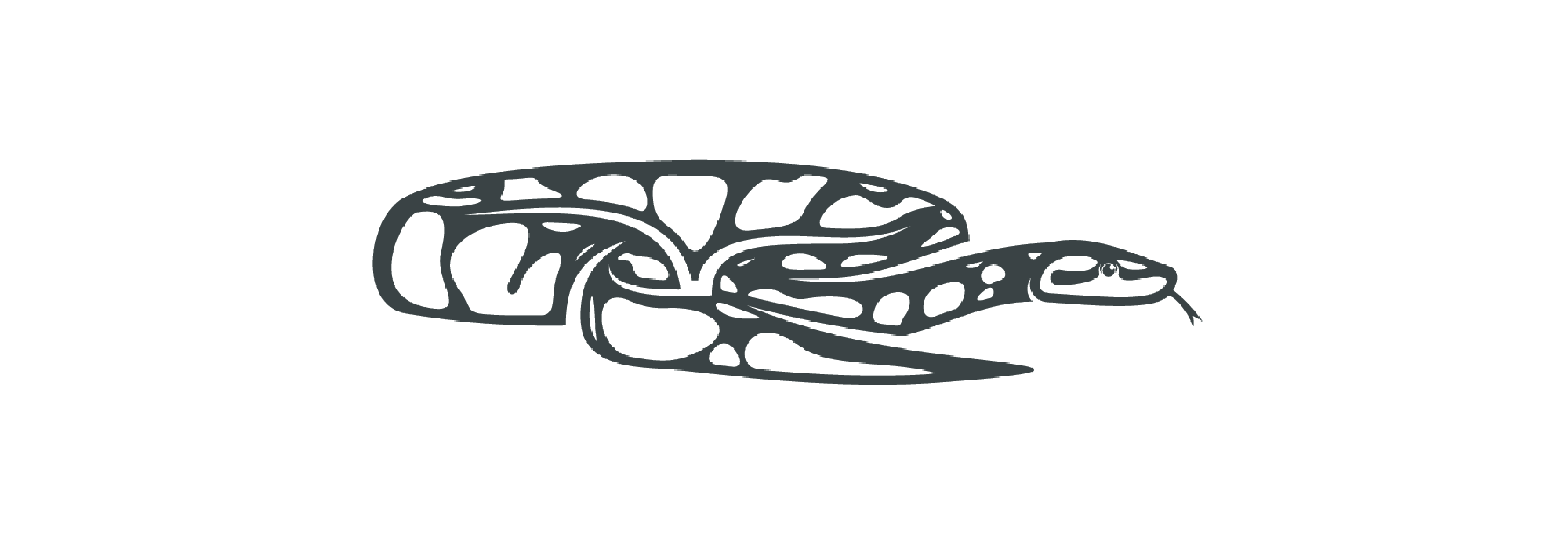
Python can be used to schedule the automated execution of preprogrammed tasks using cron. But before we dive into exactly how to automate the execution of such Python scripts, let's quickly discuss cron and Python.
About Python
Python is an extremely popular and general-purpose computer coding and programming language, which has been around since 1991. So we'll keep this brief, as you're probably well aware of what Python is.
Python is versatile, being compatible for use with many computer and programming platforms. Python can be used on Mac, Windows, Raspberry Pi, Linux, Unix, and much more.
Python can perform a vast array of activities. You can use Python to develop software and websites, perform complex mathematics, for system scripting, develop web applications, process large caches of data, facilitate rapid prototyping of programs, and much more.
The Python coding language has a user-friendly and straightforward syntax that is similar to the English language. So software developers can code programs in Python with fewer lines of code and greater efficiency of use. And Python can be treated in a functional, object-oriented, or procedural manner.

What is Cron?
Cron is an 'old-school' computer programming language that was first conceptualized in 1977. Cron is the industry-standard code for programming repetitive task assignments on predetermined schedules.
Cron is a 'daemon,' which is a phrase in programming world for a background utility. Cron can be used to schedule activities in a computer program as repetitive, regularly scheduled, or one-time task events.
Cron can easily command a system to schedule tasks, like automatically creating a spreadsheet or sending a mass email, for example, on specific and predetermined times and dates.
You can use Cron syntax on a variety of operating systems. In addition to macOS environments, Cron is the standard daemon used to operate “*nix” based computer systems (Unix, Linux, etc.).
Cron logic is also frequently used for scheduling in non-OS software, such as Zuar's Runner data pipeline solution. Using cron scheduling, Runnner can automate many tasks such as pulling data from other software, manipulating data, etc.
The cron daemon is very popular with businesses, large corporations, manufacturing concerns, and bureaucratic offices because it makes it easy to modify existing databases, initiate tasks on predetermined dates and times, and automatically program repetitive tasks.
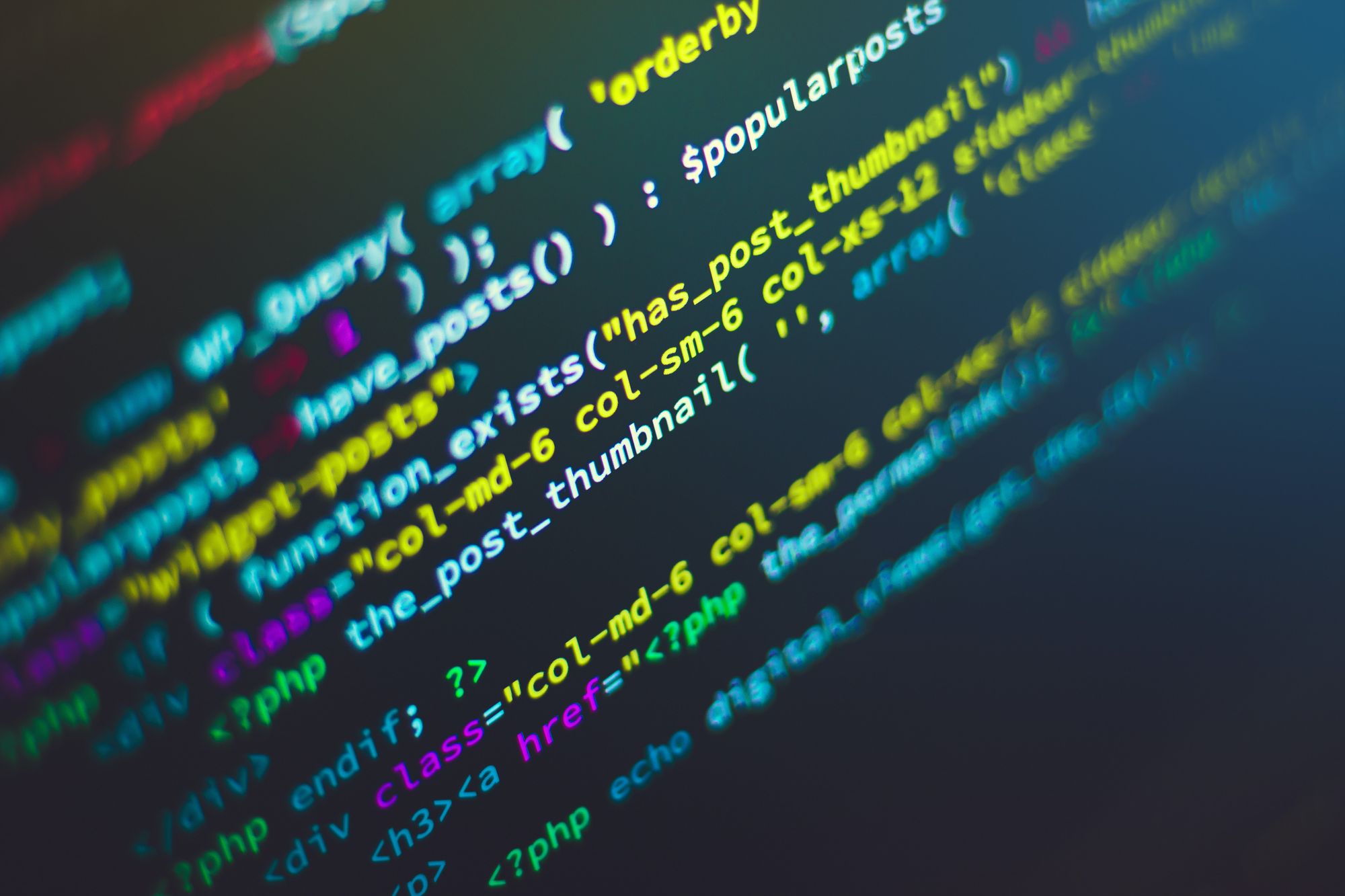
Components of a Cron Job
To better understand how to execute Python computer scripts automatically, we need to understand the components of cron:
- The script that the system executed or calls.
- Crontab is an abbreviated version of the term 'Cron Table'. It is a file containing the cron schedule to be run, and the the commands used to automate processes and functions.
- The action/output of the script.

Cron’s Six Command Fields
When inputting data to crontab for a cron job, you will use six command fields:
- The first five of these input command fields instruct the program when to initiate the scheduled activity, according to the integers you specify (or asterisks to fill unneeded fields).
- The sixth field is where you specify the command that will run at the specified time.
For purposes of context, the six command fields in a cron program can be visualized like this: * * * * * *
- First Command Field: the exact minute the crontab command is initiated. So, numbers ranging between 0 to 59 would be inputted.
- Second Command Field: equates to the exact hour of the command initiation. The numbers in this field would range between 0 to 23.
- Third Command Field: input the day of the month that the command would be executed. So, you would input a number between 1 to 31.
- Fourth Command Field: input the month of the year, so it would be a number between 1 to 12.
- Fifth Command Field: input an integer representing one of the days of the week. The numbers include 0 to 6, with '0' representing Sunday, '1' representing Monday, and so on.
- Fifth Command Field: the command sequence to initiate the cron job is inputted.
Command fields that you want the system to ignore should have an asterisk.
Here's an example:
23 18 * * 2/mydir/myprogram
This crontab tells a cron job to execute a recurring task every Tuesday at 6:23 PM. The third and fourth command fields represent the day of the month and month of initiation.
Once this makes sense to you, save time and brain power by utilizing free web tools that can help you generate this syntax.
There are special cases where you can use a keyword instead of the five-part command. For example, you can specify @daily in place of 0 0 * * * as a shortcut that's also a little more intuitive/obvious when reviewing existing jobs.
Other options include...
- @yearly
- @monthly
- @hourly
- @reboot (to launch a command when the system is booting)

How to Create a Cron Job
First, create your Python script. Then, open the system terminal your working with.
To access crontab, input 'crontab -e' (one will be created if it doesn't already exist). Then enter 'i' to initiate the edit mode, and proceed to input your schedule command.
Here is a sample schedule command for you to look over:
* * * * * cd/Users/user.name/Automation && /usr/bin/python test_cron.py
Press the 'ESC' tab to then exit edit mode. Input ':wq' to create and write your crontab. If you need to delete the whole crontab, run 'crontab -i'.
If you need to delete a single cronjob, run 'crontab -e,' press 'i,' then press 'dd' and then press ':wq' to write the file.
Let’s break down the example:
- * * * * * – the crontab command telling the script to run on a schedule of every minute
- cd – represents the command telling the crontab program where to locate the executable file is located
- /Users/user.name/Automation – indicates where the Python script can be found
- & & – separates the script command and the script name
- /usr/bin/python – denotes where the initiated Python script is located
- test_cron.py – is just a sample name for the script

Help for More Complex Data Issues
To gain a base understanding of Python, check out this free basic course offered by Coursera.
If your organizations has data issues that you can use some help with, please don't hesitate to reach out to Zuar.
- Zuar offers a wide assortment of data services, provided by certified experts
- Access more advanced data automation using our Runner data pipeline solution
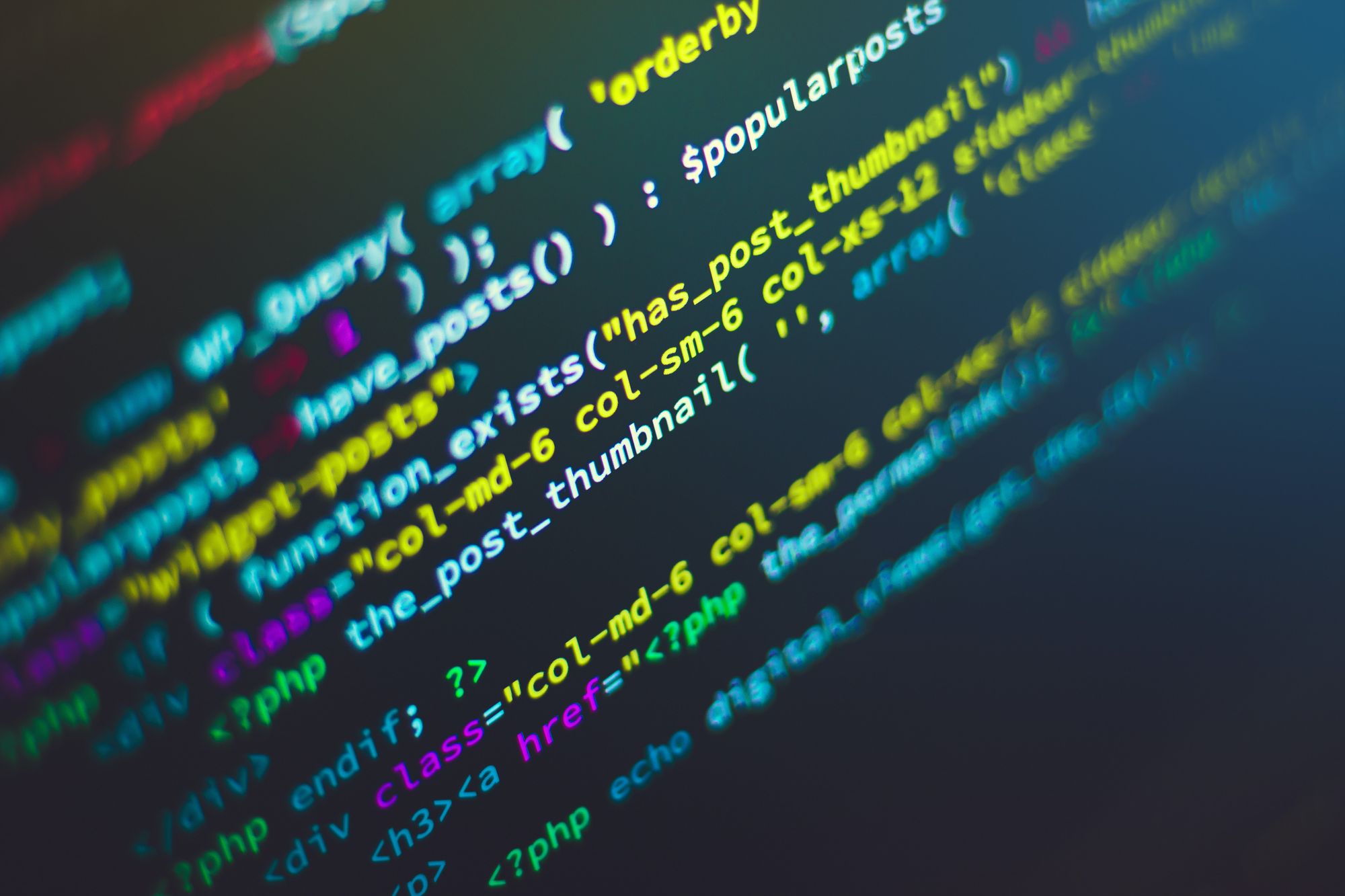
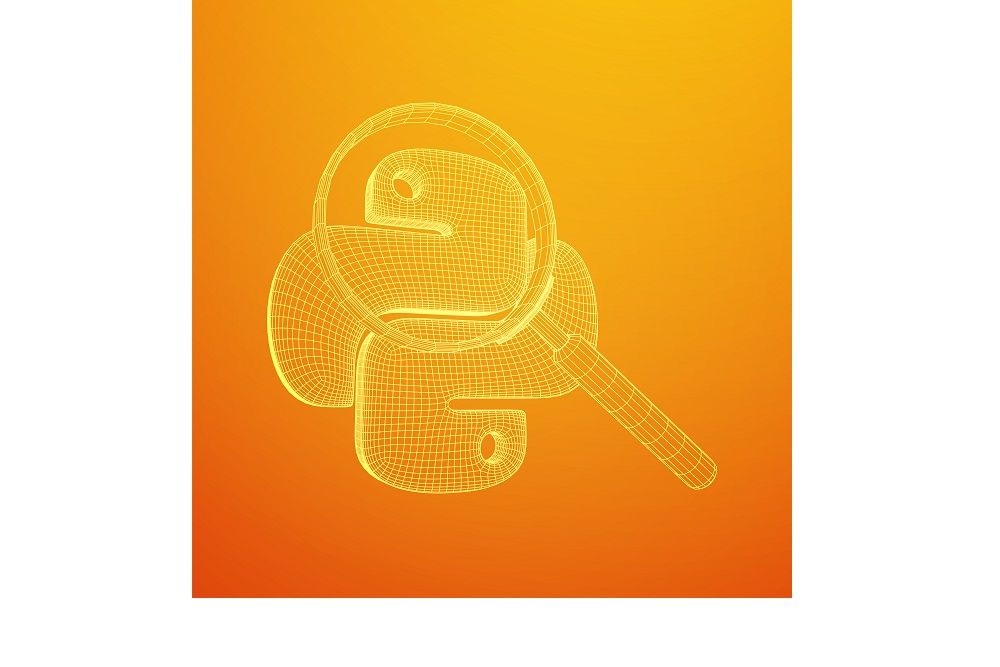
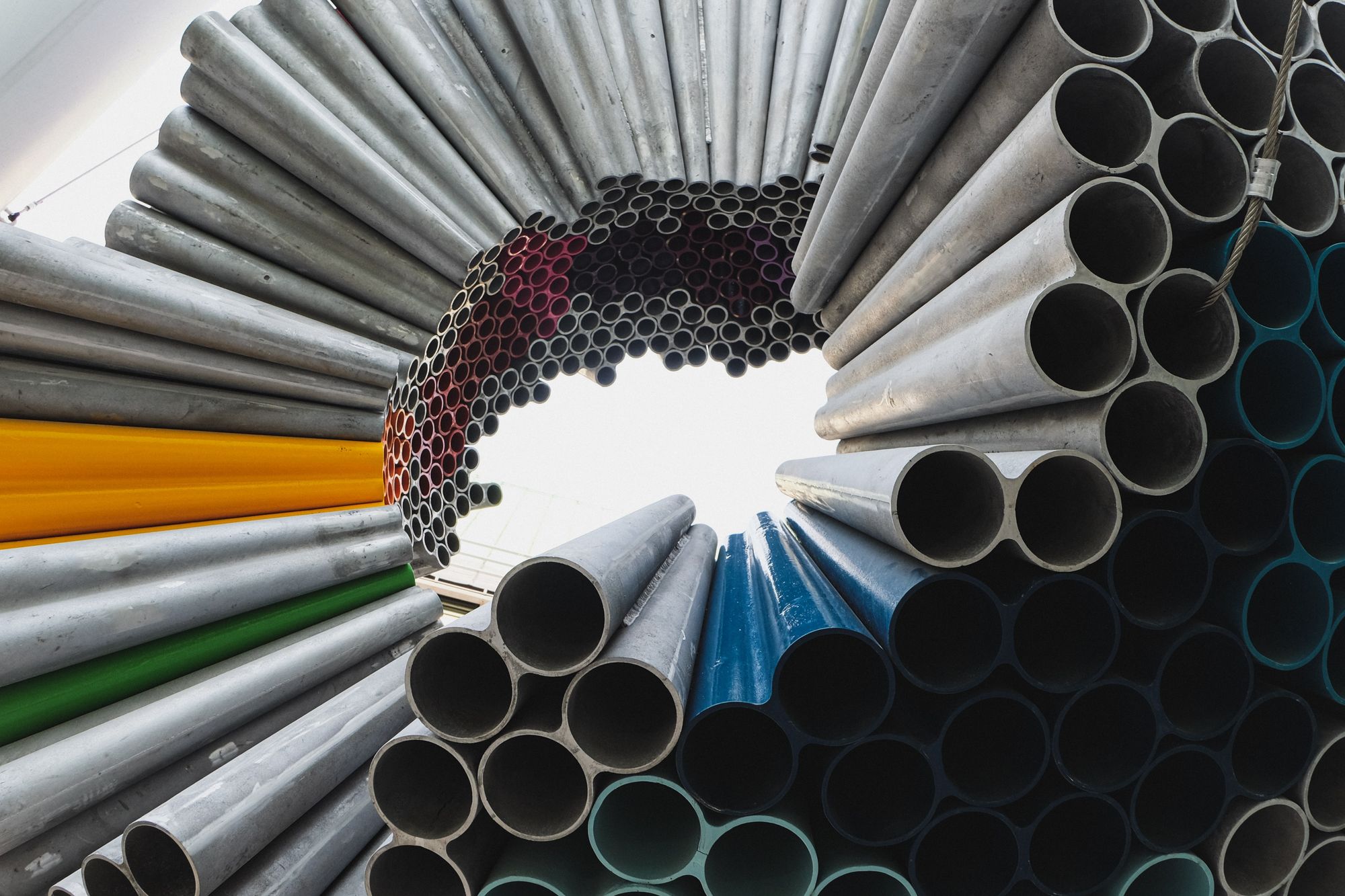