How to Embed amCharts Into a Web Page
In this article you'll see several methods for embedding an amCharts chart into a web page.
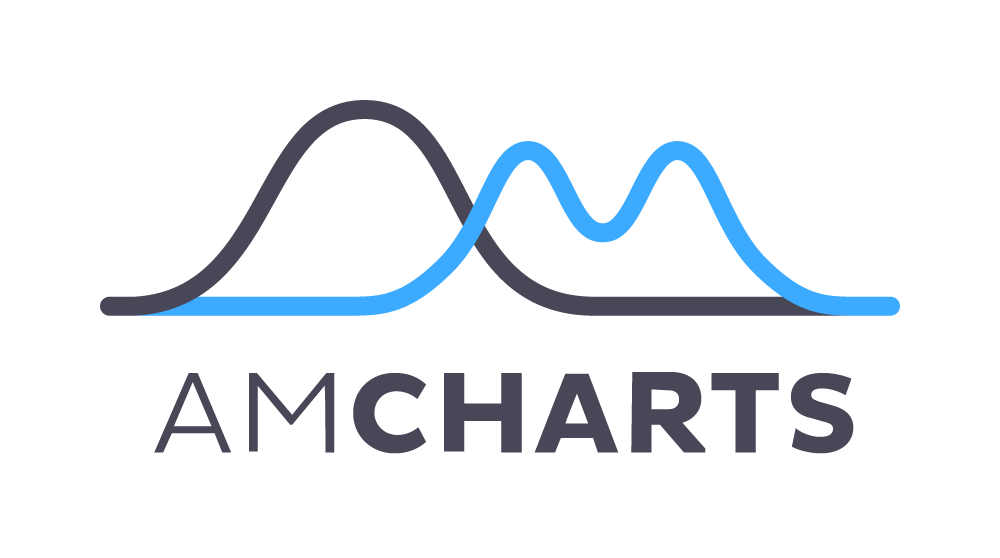
In this blog post, we show several methods for embedding an amCharts chart into a web page.
Here's the simple amCharts pie chart we will be creating: